7. Reading and plotting with Iris¶
7.1. Synopsis¶
- Use Iris to read and plot spherical data in only a few lines
7.2. Iris¶
We now turn to the package Iris whose documentation you can find at https://scitools.org.uk/iris/docs/latest/.
Install with
conda install -c conda-forge iris
although you should check their documentation for other dependencies such as cartopy (we’ve used the critical ones in earlier notebooks so if you’ve been following along you should be alright).
Here’s the usual import cell which imports both iris
and some plotting modules provided within Iris:
[1]:
import iris
import iris.plot
import iris.quickplot
import cartopy.crs
import matplotlib.pyplot as plt
In terms of what we’ve already covered, Iris plays the role of the netCDF4 and matplotlib.pyplot packages combined, much like xarray. It allows us to open a dataset and plot it with minimal intermediate steps.
First, we’ll open the same dataset that we accessed before using netCDF4.Dataset()
and xarray.open_dataset()
but this time with iris.load_cube()
:
[2]:
tos = iris.load_cube('http://esgf-data2.diasjp.net/thredds/dodsC/esg_dataroot/CMIP6/CMIP/MIROC/MIROC6/1pctCO2/r1i1p1f1/Omon/tos/gn/v20181212/tos_Omon_MIROC6_1pctCO2_r1i1p1f1_gn_330001-334912.nc')
C:\Users\ajadc\Miniconda3\envs\py3\lib\site-packages\iris\fileformats\cf.py:798: UserWarning: Missing CF-netCDF measure variable 'areacello', referenced by netCDF variable 'tos'
warnings.warn(message % (variable_name, nc_var_name))
If you see pink-background warnings, don’t worry - that’s because Iris sees a reference to a variable (areacello) that is in a different file/location. The dataset, or more precisely the “cube of data” was opened.
Note: we used iris.load_cube()
but there is also an iris.load()
. iris.load()
loads a dataset, more like xarray.open_dataset()
and would have returned a list with one element corresponding to the result of iris.load_cube()
.
[3]:
tos
[3]:
Sea Surface Temperature (degC) | time | -- | -- |
---|---|---|---|
Shape | 600 | 256 | 360 |
Dimension coordinates | |||
time | x | - | - |
Auxiliary coordinates | |||
latitude | - | x | x |
longitude | - | x | x |
Attributes | |||
Conventions | CF-1.7 CMIP-6.2 | ||
DODS_EXTRA.Unlimited_Dimension | time | ||
_ChunkSizes | [ 1 256 360] | ||
activity_id | CMIP | ||
branch_method | standard | ||
branch_time_in_child | 0.0 | ||
branch_time_in_parent | 0.0 | ||
cmor_version | 3.3.2 | ||
comment | Temperature of upper boundary of the liquid ocean, including temperatures... | ||
creation_date | 2018-11-30T13:31:08Z | ||
data_specs_version | 01.00.28 | ||
experiment | 1 percent per year increase in CO2 | ||
experiment_id | 1pctCO2 | ||
external_variables | areacello | ||
forcing_index | 1 | ||
frequency | mon | ||
further_info_url | https://furtherinfo.es-doc.org/CMIP6.MIROC.MIROC6.1pctCO2.none.r1i1p1f... | ||
grid | native ocean tripolar grid with 360x256 cells | ||
grid_label | gn | ||
history | 2018-11-30T13:31:08Z altered by CMOR: replaced missing value flag (-999)... | ||
initialization_index | 1 | ||
institution | JAMSTEC (Japan Agency for Marine-Earth Science and Technology, Kanagawa... | ||
institution_id | MIROC | ||
license | CMIP6 model data produced by MIROC is licensed under a Creative Commons... | ||
mip_era | CMIP6 | ||
nominal_resolution | 100 km | ||
original_name | TO | ||
parent_activity_id | CMIP | ||
parent_experiment_id | piControl | ||
parent_mip_era | CMIP6 | ||
parent_source_id | MIROC6 | ||
parent_time_units | days since 3200-1-1 | ||
parent_variant_label | r1i1p1f1 | ||
physics_index | 1 | ||
product | model-output | ||
realization_index | 1 | ||
realm | ocean | ||
source | MIROC6 (2017): | ||
aerosol | SPRINTARS6.0 | ||
atmos | CCSR AGCM (T85; 256 x 128... | ||
source_id | MIROC6 | ||
source_type | AOGCM AER | ||
sub_experiment | none | ||
sub_experiment_id | none | ||
table_id | Omon | ||
table_info | Creation Date:(06 November 2018) MD5:0728c79344e0f262bb76e4f9ff0d9afc | ||
title | MIROC6 output prepared for CMIP6 | ||
tracking_id | hdl:21.14100/636faabd-555e-414a-bbba-e251ce0f2936 | ||
variable_id | tos | ||
variant_label | r1i1p1f1 | ||
Cell methods | |||
mean where sea | area | ||
mean | time |
This is the same information that we saw with netCDF4.Dataset or xarray.Dataset objects and is nicely displayed (if we had used print(tos)
we would see an ascii form of the above html).
Notice that the coordinates are indicated as 2D and there is no mention of the 1D spatial dimension variables. This is great because it means Iris has properly interpreted the metadata following the CF convention and knows that the data resides an a non-trivial grid.
Do we still have to use cartopy (as with netCDF4 or xarray) to handle spherical coordinates?
[4]:
iris.plot.pcolormesh( tos[0] );
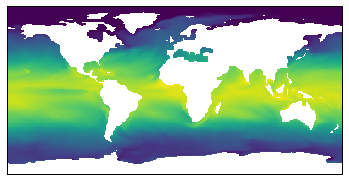
This has plotted things correctly and seems to be in a Plate-Carree (lat-lon) projection. Notice that this call to pcolormesh() did not add labels or a colorbar automatically. For that, Iris provide an alternative to their plot module called quickplot. Here’s an examples also using a different cartopy projection.
[5]:
ax = plt.axes(projection=cartopy.crs.Robinson());
iris.quickplot.pcolormesh( tos[0] );
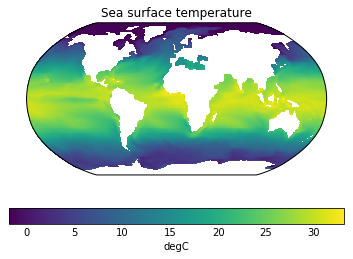
Unlike xarray, the time of the dataset was not added to the title but otherwise the plot is fairly complete and useful.
Notice that the transform=
argument to pcolormesh()
is not needed because Iris provides that information for you.
As a last test, let’s see if Iris knows about the finite-volume nature of the data and can plot the Arctic properly without a seam…
[6]:
ax = plt.axes(projection=cartopy.crs.NorthPolarStereo());
iris.quickplot.pcolormesh( tos[0], vmin=-2, vmax=8 );
w=2e6; plt.xlim(-w,w); plt.ylim(-w,w); # Zoom into Arctic
plt.gca().coastlines();
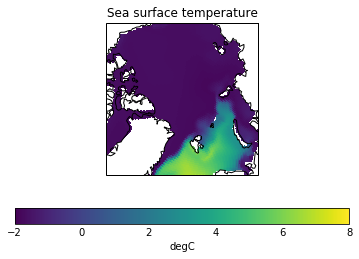
7.3. Summary¶
- Iris is a high-level layer that understands the CF convention in netCDF files.
- Iris can plot ocean-model data correctly out-of-the-box!